XSS: Developer’s Perspective explained with Simple Examples
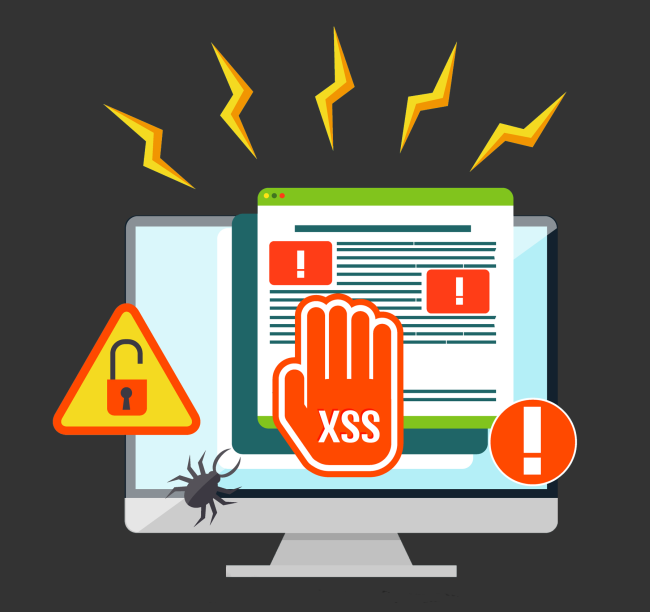
Developers spend plenty of their time fixing bugs and errors to keep their code running. They have to keep a track of the ever-changing frameworks, updates, and tools. Vulnerability handling and prevention should be a part of the development process when security is the utmost priority. Hence understanding these bugs and vulnerabilities from a developer’s viewpoint can be a productive strategy for bug hunting and research.
Cross-Site Scripting (XSS)
Cross-Site Scripting, in simple words, is a client-side vulnerability that allows the adversaries to send malicious code and data through user input that may include a form or a comment box.
XSS has consistently found its place in the OWASP TOP 10 list. I found various reasons for the same:
Some browsers (like Firefox) still don’t implement the XSS filters and do not have the X-XSS-Protection header enabled.
Sometimes the auditor may not block it because a bypass is identified after some implementations.
Still, due to the false positives negatives, some payloads may still be undetected.
I have coded two web apps first will an XSS vulnerability and the second with a cookie injection.
XSS vulnerable contact form
In this web application, I have coded a simple contact form with GET parameter. The code is available at the git repo.
This has three fields- Name, Email and message.
Using the GET parameter the PHP part looks like this:
<?php
if ($_GET)
{
echo "<br>";
echo 'Your Input:';
echo "<br>";
echo $_GET["name"];
echo "<br>";
echo $_GET["email"];
echo "<br>";
echo $_GET["message"];
}
?>
This form validates the input i.e. it shows it after it is submitted.
Now, I will try to put an alert through the script tags in the Name field.
<script>alert("vulnerable!!!")</script>
After submitting, I see an alert:
This is a reflected XSS attack, I was able to display an alert through the form input.
Prevention
This attack can be prevented by filtering out special characters like < and / through the PHP script. This code snippet can be added to the php part:
function test_input($data) {
$data = trim($data);
$data = stripslashes($data);
$data = htmlspecialchars($data);
return $data;
}
Cookie injection vulnerable Login Page
A little background on cookies: you must be familiar with the “Remember me” option in login pages. These pages tend to store user information and preferences in the form of cookies. These cookies can be edited and hence attackers may try to steal confidential user information through cookie injections.
Privilege escalation (logging in as the administrator user ) can be implemented in the application to uncover code injection vulnerabilities.
This web-app is a simple login page. You can access it at the same git repo.
I try entering some random username and password but the login fails.
In the credentials.conf file I have added the user and admin credentials.
So I now try logging in as user.
Successful login! Now I will login with administrative id-pass
I again login as user and try to escalate it’s privilege by storage Web Developer>Storage Editor in Firefox. (Or just press Shift+F7)
This opens the storage editor and I change the value of userrole to admin.
Reload the page and I am logged in as admin!
In a real-time attack logging in as admin can expose quite juicy information to the attacker compromising the security of the application.
Prevention
Following some simple steps like installing an SSL certificate, security plugins and an effective antivirus and keeping everything updated can reduce the possibility of a cookie injection on a website.
Conclusion
XSS vulnerabilities have been prevailing for more than a decade now, hence it is still found in web applications and exploited by the attackers. Validating and sanitizing the user input are essential aspects of web security and must be incorporated in the applications.
This blog post was quite a bit of a challenge for me as I am new to developing web applications. Thanks, Harshit for the help with PHP coding!
Thank you for reading :)